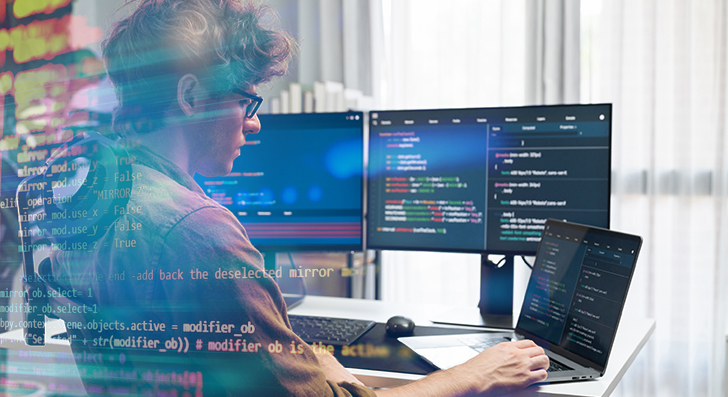
Scalability implies your software can cope with progress—much more users, additional data, and more website traffic—with no breaking. As being a developer, building with scalability in your mind saves time and strain later. Right here’s a transparent and functional guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a thing you bolt on afterwards—it should be aspect of one's system from the beginning. Quite a few applications fall short when they increase rapidly because the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your method will behave stressed.
Start by developing your architecture to generally be flexible. Keep away from monolithic codebases where by every little thing is tightly related. Instead, use modular style and design or microservices. These patterns break your app into scaled-down, unbiased components. Every single module or company can scale on its own with out influencing the whole method.
Also, think of your databases from working day one. Will it require to deal with 1,000,000 users or merely a hundred? Choose the ideal style—relational or NoSQL—based upon how your information will mature. System for sharding, indexing, and backups early, even if you don’t have to have them still.
Yet another important position is to avoid hardcoding assumptions. Don’t produce code that only operates less than recent ailments. Think about what would occur Should your consumer base doubled tomorrow. Would your app crash? Would the database decelerate?
Use design patterns that assistance scaling, like message queues or event-pushed units. These help your application take care of far more requests devoid of receiving overloaded.
If you Create with scalability in mind, you're not just making ready for fulfillment—you're decreasing future problems. A very well-prepared program is easier to take care of, adapt, and improve. It’s superior to organize early than to rebuild later on.
Use the best Database
Choosing the right databases is actually a important part of setting up scalable apps. Not all databases are developed a similar, and utilizing the Incorrect you can sluggish you down or perhaps induce failures as your app grows.
Begin by being familiar with your knowledge. Could it be very structured, like rows in a desk? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically robust with interactions, transactions, and consistency. They also guidance scaling strategies like read replicas, indexing, and partitioning to manage more targeted traffic and information.
If the information is much more flexible—like person activity logs, product or service catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling huge volumes of unstructured or semi-structured details and may scale horizontally additional quickly.
Also, consider your read through and generate patterns. Do you think you're doing a lot of reads with less writes? Use caching and skim replicas. Are you currently dealing with a significant compose load? Check into databases that can manage significant generate throughput, or perhaps function-dependent details storage methods like Apache Kafka (for short term data streams).
It’s also intelligent to Feel forward. You might not will need advanced scaling attributes now, but selecting a database that supports them implies you gained’t need to have to switch later.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your knowledge determined by your entry styles. And generally observe databases general performance as you grow.
In short, the proper database depends on your application’s composition, velocity desires, And just how you be expecting it to increase. Choose time to select sensibly—it’ll help save a great deal of difficulties later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single modest hold off adds up. Poorly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s vital that you Develop efficient logic from the beginning.
Start off by creating clean, easy code. Avoid repeating logic and take away everything needless. Don’t choose the most advanced Resolution if a simple a person performs. Keep your capabilities limited, centered, and easy to check. Use profiling equipment to locate bottlenecks—sites in which your code requires far too extended to operate or employs an excessive amount of memory.
Upcoming, check out your database queries. These generally slow points down over the code alone. Ensure Each individual query only asks for the info you actually need to have. Steer Developers blog clear of SELECT *, which fetches almost everything, and instead decide on unique fields. Use indexes to speed up lookups. And prevent performing too many joins, Primarily across massive tables.
For those who recognize the same knowledge remaining requested over and over, use caching. Retail store the results briefly working with applications like Redis or Memcached which means you don’t should repeat highly-priced operations.
Also, batch your database functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more successful.
Make sure to exam with large datasets. Code and queries that perform wonderful with one hundred records may crash after they have to manage one million.
Briefly, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when desired. These steps assist your application stay clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's to manage far more end users plus much more website traffic. If all the things goes as a result of a person server, it will eventually immediately become a bottleneck. That’s exactly where load balancing and caching come in. Both of these applications enable maintain your app quickly, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server executing all the do the job, the load balancer routes people to diverse servers determined by availability. This implies no one server receives overloaded. If one particular server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing facts briefly so it can be reused promptly. When consumers request the exact same data again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. It is possible to serve it with the cache.
There are two popular forms of caching:
one. Server-aspect caching (like Redis or Memcached) stores knowledge in memory for fast entry.
2. Customer-facet caching (like browser caching or CDN caching) merchants static data files near to the user.
Caching lessens database load, enhances velocity, and can make your application a lot more economical.
Use caching for things that don’t transform frequently. And constantly make sure your cache is up-to-date when data does adjust.
In brief, load balancing and caching are uncomplicated but potent instruments. Alongside one another, they help your application tackle much more end users, continue to be quick, and Get well from complications. If you plan to increase, you would like each.
Use Cloud and Container Applications
To construct scalable programs, you require tools that let your app increase quickly. That’s where cloud platforms and containers come in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to get components or guess long run potential. When targeted visitors increases, you can add much more sources with only a few clicks or instantly making use of automobile-scaling. When website traffic drops, you may scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection instruments. It is possible to target constructing your app rather than managing infrastructure.
Containers are another vital Software. A container deals your application and every little thing it has to run—code, libraries, settings—into one device. This causes it to be simple to move your application amongst environments, out of your notebook to your cloud, with no surprises. Docker is the most popular tool for this.
Once your app uses many containers, equipment like Kubernetes assist you to control them. Kubernetes handles deployment, scaling, and Restoration. If just one element of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate aspects of your app into services. It is possible to update or scale components independently, which happens to be great for performance and dependability.
In short, employing cloud and container resources means you may scale quick, deploy quickly, and recover promptly when issues transpire. If you would like your application to grow with no restrictions, commence applying these resources early. They help save time, reduce chance, and assist you remain centered on setting up, not repairing.
Observe Every little thing
When you don’t monitor your application, you gained’t know when points go Incorrect. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make improved decisions as your app grows. It’s a critical part of developing scalable programs.
Start out by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you accumulate and visualize this details.
Don’t just monitor your servers—monitor your app as well. Keep watch over how long it will take for consumers to load webpages, how often mistakes take place, and the place they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or a service goes down, you should get notified straight away. This allows you take care of difficulties rapidly, typically just before customers even notice.
Checking is likewise practical any time you make alterations. Should you deploy a brand new feature and find out a spike in problems or slowdowns, you are able to roll it again in advance of it triggers genuine destruction.
As your application grows, visitors and data raise. Without having monitoring, you’ll miss out on signs of hassle right up until it’s as well late. But with the ideal equipment in place, you keep in control.
In short, monitoring helps you maintain your app reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your process and ensuring it really works nicely, even stressed.
Final Feelings
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By building very carefully, optimizing sensibly, and using the appropriate applications, you'll be able to Develop applications that mature easily devoid of breaking under pressure. Commence compact, Believe massive, and Establish wise.